x = x + α(x0 - x)This is also equivalent to
x = (1-α)x + αx0This can be used for easing out, not easing in. In this post I'll demonstrate a simple method for easing in&out simultaneously.
Suppose that the current positin of the movie clip is at x0, and the final (target) position is at x1. We need to find a function which takes x0, x1, t (current time), and tf (total time) as parameters and returns the expected position at time t.
f(x0, x1, t, tf)We can use sine function for this purpose. The below figure is the sine function between -π/2 and π/2.
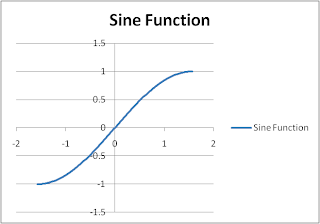
x = f(x0, x1, t, tf) = (x1-x0) sin((t/tf - 0.5)*π)+1)/2In ActionScript 2.0, you can use the following code where box_mc is the movieclip instance you want to move with ease.
You can download the source code. Checkout the following example. (Google Sites fixes the problem with flash files it should work now)
var t = 0;
var tf = 40;
var x0 = 0;
var x1 = 500;
onEnterFrame = function ()
{
if (t<tf)
{
box_mc._x = (x1-x0) * (Math.sin((t/tf - 0.5)*Math.PI)+1)/2;
t++;
}
}
Also check out TweenLite which is also used in Gaia Flash Framework. You will have lots of tweening types like these ones, and you will be able to use them with just a single line of code. It has also very small fingerprint.
Keep flashing...
No comments:
Post a Comment